Bulk Conversations Transcript Export
Overview
To export all your conversations, we recommend looping through all the pages of the list conversations endpoint to obtain all the conversation ID's. Then, retrieve each individual conversation by iterating through your list. Grab the conversation object as a JSON or download the transcript as a string.
Application Set Up
Create a Drift app in order to generate an OAuth Access Token. The OAuth Access Token is required to make any requests to your Drift organization through the API.
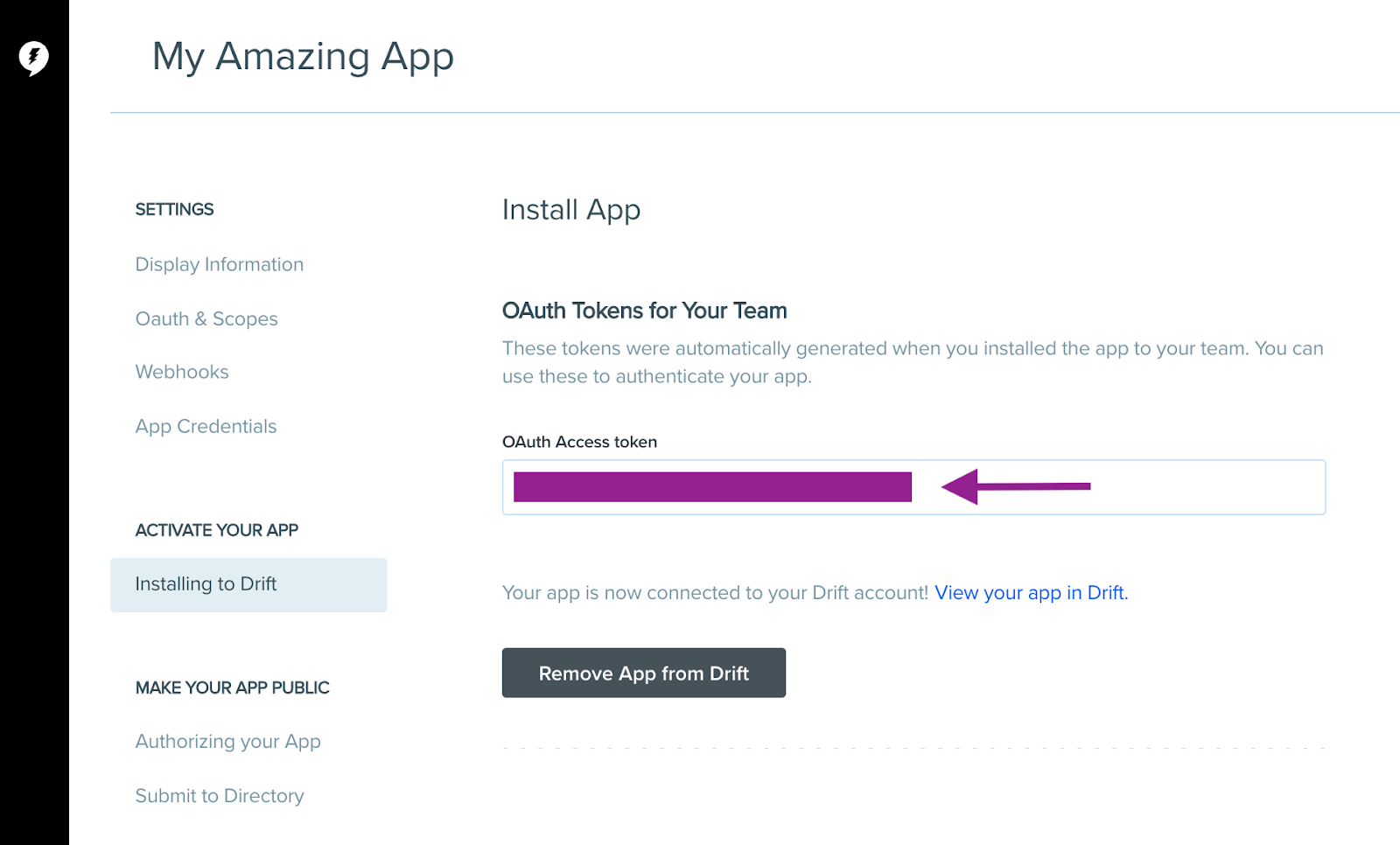
Required Auth Scopes:
conversation_read
user_read
contact_read
Script Set up
The script is backend Javascript using Node.js environment. The same concept can be applied or hosted on any serverside platform (e.g., Python, Ruby, etc)
- Initiate Drift OAuth Access Token
We are using 'dotenv' that loads environment variables from a .env file into process.env.
require('dotenv').config()
const DRIFT_AUTH_TOKEN = process.env.DRIFT_AUTH_TOKEN
- Obtain the total list of conversations returned from the Data object in the list conversations endpoint.
Query Param | Type | Description |
---|---|---|
limit (optional) | int | Max number of conversations to retrieve (max 50, default 25). |
next (optional) | int | Cursor param used for pagination (explained below) |
statusId (optional, repeatable) | int | Return only conversations that match the provided status code. Uses the following values: 1: OPEN 2: CLOSED 3: PENDING |
// retrieve all conversations over a set period of time
require('dotenv').config()
const DRIFT_AUTH_TOKEN = process.env.DRIFT_AUTH_TOKEN
const axios = require('axios'); // using Axios for HTTP requests
const url = 'https://driftapi.com/reports/conversations'
const headers = {
'Authorization': `Bearer ${DRIFT_AUTH_TOKEN}`,
'Content-Type': 'application/json'
}
const now = new Date().getTime(); // Defines now time to run a report within a time range
// review other filtering options here - https://github.com/Driftt/Drift-Bulk-Export-Transcript/blob/master/Drift/listConvoIds.js
// Current filter is:
// - Conversations is close
// - Has a chat Agent [bot or user]
// - List of metrics to export ["createdAt","lastAgentId","endUserId","timeToCloseMillis","numAgentMessages","numBotMessages","numEndUserMessages"]
const data = JSON.stringify({
"filters": [
{
"property": "status",
"operation": "IN",
"values": [
"closed"
]
},
{
"property": "lastAgentId",
"operation": "HAS_PROPERTY"
},
{
"property": "updatedAt",
"operation": "BETWEEN",
"values": [
//now-(1000*60*60*24*10), //ensure this aligns with timer settings to run app.js
// now
"2021-xx-xx",
"2021-xx-xx"
]
}
],
"metrics": [
"createdAt",
"lastAgentId",
"endUserId",
"timeToCloseMillis",
"numAgentMessages",
"numBotMessages",
"numEndUserMessages"
]
});
const convoReport = async () => {
return axios
.post(url, data, {headers: headers})
.then((res) => {
// Track total number of conversations returns that checks the filter above
console.log("Number of Drift conversations returned: " + res.data.count);
let fullResponse = (res.data);
let conversations = [];
if(fullResponse.count == 0) {
return "no new conversations"; // Call out if there are no new conversations
};
// Loop through the list and hold into each conversationId
fullResponse.data.forEach(conversation => {
conversations.push({
conversationId: conversation.conversationId,
metrics: conversation.metrics
})
})
return conversations
})
// Standard catch error
.catch(err => {
console.log("ERR HITTING URL ---> " + err.config.url);
console.log("ERR CODE ---> " + err.response.status);
console.log("ERR DATE ---> " + err.response.headers.date);
console.log("ERR MSG ---> " + err.message);
return "Error retrieving conversations."
})
}
module.exports = {
convoReport
};
3.A Loop through each conversationId to pull down each message body for each individual conversation.
require('dotenv').config()
const DRIFT_AUTH_TOKEN = process.env.DRIFT_AUTH_TOKEN;
const axios = require("axios");
const baseUrl = "https://driftapi.com/conversations/";
const headers = {
Authorization: `Bearer ${DRIFT_AUTH_TOKEN}`,
"Content-Type": "application/json",
};
// Use this endpoint to get more detailed information about a particular conversation, such as participant ids, tags, related playbooks, etc.,
const getConversation = async (conversationId) => {
return axios
.get(baseUrl + conversationId, { headers: headers })
.then((res) => {
let convoObject = res.data; // Store conversation object
return convoObject;
})
.catch((err) => {
console.log("Error fetching conversation data for conversation " + conversationId);
console.log("ERR HITTING URL ---> " + err.config.url);
console.log("ERR CODE ---> " + err.response.status);
console.log("ERR DATE ---> " + err.response.headers.date);
console.log("ERR MSG ---> " + err.message);
return "Error"
});
};
module.exports = {
getConversation,
};
3.B. If you prefer to export the transcript instead of the messages body, loop through each individual conversation transcript.
require('dotenv').config()
const DRIFT_AUTH_TOKEN = process.env.DRIFT_AUTH_TOKEN;
const axios = require("axios");
const baseUrl = "https://driftapi.com/conversations/";
const headers = {
Authorization: `Bearer ${DRIFT_AUTH_TOKEN}`,
"Content-Type": "application/json",
};
// Returns the formatted transcript of the Conversation with id equal to the given conversationId as a string object.
// Requires conversation_read scope in your authorized app - https://dev.drift.com/apps.
const getTranscript = async (conversationId) => {
return axios
.get(baseUrl + conversationId + "/transcript", { headers: headers })
.then((res) => {
let transcript = res.data // The response object will be a formatted string of the entire transcript
return transcript;
})
.catch((err) => {
console.log("Error fetching conversation data for conversation " + conversationId);
console.log("ERR HITTING URL ---> " + err.config.url);
console.log("ERR CODE ---> " + err.response.status);
console.log("ERR DATE ---> " + err.response.headers.date);
console.log("ERR MSG ---> " + err.message);
return "Error"
});
};
module.exports = {
getTranscript,
};
Perfect! Okay, one last step.
- Create a CSV file with the proper columns using your preferred CSV generator library
// Documentations - https://www.npmjs.com/package/csv-writer
//Writes objects/arrays into a CSV string into a file.
const createCsvWriter = require("csv-writer").createObjectCsvWriter;
const date = new Date(); // Set time to differentiate exported file's name
const csvWriter = createCsvWriter({
path: "./ExportFiles/" + "DriftCSV-" + date + ".csv",
//Array of objects (id and title properties) or strings (field IDs).
//A header line will be written to the file only if given as an array of objects.
header: [
{ id: "convo_id", title: "convo_id" },
{ id: "assignee_id", title: "assignee_id" },
{ id: "link_to_full_conversation", title: "link_to_full_conversation" },
{ id: "company_name", title: "company_name" },
{ id: "updatedat_date", title: "updatedat_date" },
{ id: "createdat_date", title: "createdat_date" },
{ id: "participant", title: "participant" },
{ id: "transcriptObject", title: "transcriptObject" },
{ id: "total_messages", title: "total_messages" },
],
});
const csvCreate = async (interactions) => {
const body = interactions;
// Write collected data into the CSV file
return csvWriter
.writeRecords(body)
.then(() => console.log("Data uploaded into csv successfully"))
.catch((err) => {
console.log("Error exporting conversations to CSV.");
return "Error exporting conversations to CSV.";
});
};
module.exports = csvCreate;
Want to see a Bulk Export in action?
Head over to Bulk Conversations Transcript Export and see what it looks like!
Awesome! You should have a working Drift script sample- feel free to change any of the exported attributes/data or modify the code as you like.
Updated over 2 years ago